Hallo. Ich habe das Problem, das ich als Teil meines Codes eine bzw. zwei Methoden habe, welche die Daten aus CSV Dateien lesen sollen, welche sich in der Projektstruktur befinden (siehe Bild) und später auch in der runnable JAR bleiben sollen. Diese Daten werden dann später im Programm genutzt um Geographische Namen zu referenzieren. Ich habe bereits das eine oder andere Debugging probiert. Es scheint als wird die Datei bei Programmstart aus Eclipse nicht gefunden oder bei der Übergabe vom InputStream zum Reader funktioniert etwas nicht. Ich habe auch schon einige andere Ordnerstrukturen verwendet um auf die Dateien zuzugreifen. Fällt jemandem was auf, wo der Fehler liegen könnte. Vielen Dank schon mal. Ich hoffe ich habe den Code richtig dargestellt (ist mein erster Beitrag).
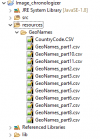
private void loadGeoNames() {
for (int i = 1; i <= 11; i++) {
String filePath = "GeoNames/GeoNames_part" + i + ".csv";
System.out.println("Versuche, Datei zu laden: " + filePath);
try (InputStream inputStream = ClassLoader.getSystemClassLoader().getResourceAsStream(filePath)) {
if (inputStream == null) {
System.out.println("Ressource nicht gefunden: " + filePath);
JOptionPane.showMessageDialog(this, "GeoNames-Datei nicht gefunden: " + filePath);
continue;
}
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.startsWith("geonameid")) {
continue;
}
String[] fields = line.split(";");
if (fields.length >= 12) {
try {
String city = fields[2].trim();
String country = fields[0].trim();
String latitudeStr = fields[9].trim().replace(",", ".");
String longitudeStr = fields[10].trim().replace(",", ".");
if (!latitudeStr.isEmpty() && !longitudeStr.isEmpty()) {
try {
double latitude = Double.parseDouble(latitudeStr);
double longitude = Double.parseDouble(longitudeStr);
geoNames.add(new GeoNameEntry(city, country, latitude, longitude));
} catch (NumberFormatException e) {
continue;
}
}
} catch (Exception e) {
continue;
}
}
}
}
} catch (IOException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Fehler beim Laden der GeoNames-Datei " + filePath);
}
}
}
private void loadCountryCodes() {
String filePath = "GeoNames/CountryCode.CSV";
System.out.println("Versuche, Datei zu laden: " + filePath);
try (InputStream inputStream = ClassLoader.getSystemClassLoader().getResourceAsStream(filePath)) {
if (inputStream == null) {
System.out.println("Ressource nicht gefunden: " + filePath);
JOptionPane.showMessageDialog(this, "CountryCode-Datei nicht gefunden: " + filePath);
return;
}
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.startsWith("Country")) {
continue; // Überspringe die Kopfzeile
}
String[] fields = line.split(";");
if (fields.length >= 5) {
String countryCode = fields[0].trim();
String countryName = fields[4].trim();
countryCodes.put(countryCode, countryName);
}
}
}
} catch (IOException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Fehler beim Laden der CountryCode-Datei " + filePath);
}
}
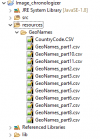
private void loadGeoNames() {
for (int i = 1; i <= 11; i++) {
String filePath = "GeoNames/GeoNames_part" + i + ".csv";
System.out.println("Versuche, Datei zu laden: " + filePath);
try (InputStream inputStream = ClassLoader.getSystemClassLoader().getResourceAsStream(filePath)) {
if (inputStream == null) {
System.out.println("Ressource nicht gefunden: " + filePath);
JOptionPane.showMessageDialog(this, "GeoNames-Datei nicht gefunden: " + filePath);
continue;
}
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.startsWith("geonameid")) {
continue;
}
String[] fields = line.split(";");
if (fields.length >= 12) {
try {
String city = fields[2].trim();
String country = fields[0].trim();
String latitudeStr = fields[9].trim().replace(",", ".");
String longitudeStr = fields[10].trim().replace(",", ".");
if (!latitudeStr.isEmpty() && !longitudeStr.isEmpty()) {
try {
double latitude = Double.parseDouble(latitudeStr);
double longitude = Double.parseDouble(longitudeStr);
geoNames.add(new GeoNameEntry(city, country, latitude, longitude));
} catch (NumberFormatException e) {
continue;
}
}
} catch (Exception e) {
continue;
}
}
}
}
} catch (IOException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Fehler beim Laden der GeoNames-Datei " + filePath);
}
}
}
private void loadCountryCodes() {
String filePath = "GeoNames/CountryCode.CSV";
System.out.println("Versuche, Datei zu laden: " + filePath);
try (InputStream inputStream = ClassLoader.getSystemClassLoader().getResourceAsStream(filePath)) {
if (inputStream == null) {
System.out.println("Ressource nicht gefunden: " + filePath);
JOptionPane.showMessageDialog(this, "CountryCode-Datei nicht gefunden: " + filePath);
return;
}
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.startsWith("Country")) {
continue; // Überspringe die Kopfzeile
}
String[] fields = line.split(";");
if (fields.length >= 5) {
String countryCode = fields[0].trim();
String countryName = fields[4].trim();
countryCodes.put(countryCode, countryName);
}
}
}
} catch (IOException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Fehler beim Laden der CountryCode-Datei " + filePath);
}
}