Ich möchte eine Komponente für Dialoge programmieren, die Aussieht wie das erste Bild. Was ich bislang habe, sieht allerdings aus wie das zweite Bild.
1.) Ich frage mich, woher der Fenster-Balken unter der Titelleiste kommt.
2.) Wie lässt sich das Look&Feel realisieren, dass man bei gedrückter Maustaste über dem gelb-schwarzen Balken das Fenster verschieben kann?
3.) Der weiße Rahmen um das Bild ist transparent, und sieht nur auf dem Screenshot weiß aus. Allerdings nimmt er immer die Farbe an von dem Fenster das darunter liegt während er aktiv ist und bewegt wird, ist also nicht wirklich transparent. wie kann ich das ändern?
4.) Wie kann ich den InternalFrame modal anzeigen lassen, während weitere InternalFrames geöffnet sind?
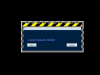
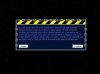
hier der Sourcecode:
1.) Ich frage mich, woher der Fenster-Balken unter der Titelleiste kommt.
2.) Wie lässt sich das Look&Feel realisieren, dass man bei gedrückter Maustaste über dem gelb-schwarzen Balken das Fenster verschieben kann?
3.) Der weiße Rahmen um das Bild ist transparent, und sieht nur auf dem Screenshot weiß aus. Allerdings nimmt er immer die Farbe an von dem Fenster das darunter liegt während er aktiv ist und bewegt wird, ist also nicht wirklich transparent. wie kann ich das ändern?
4.) Wie kann ich den InternalFrame modal anzeigen lassen, während weitere InternalFrames geöffnet sind?
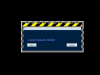
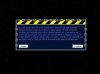
hier der Sourcecode:
Java:
package gui;
import java.awt.Color;
import java.awt.Component;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import javax.swing.JButton;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.border.Border;
/**
* Abstract Basic Class for Dialogs which are displayed centered in the
* middle of the screen in modal or non-modal mode
*
* @author Lord
*
*/
@SuppressWarnings("serial")
public class BasicDialog extends JInternalFrame {
class AttentionBorder implements Border {
public void paintBorder(Component comp, Graphics g, int left, int top, int width, int height) {
// TODO: Enforce Border do be top and down, and frame to be embedded.. then easily
// overwriting the image can be done without creating new subimages..
boolean toggle;
final int BORDER = 10, // Gibt die Höhe=Breite der Grenzen an
edge = node_topleft.getHeight(), // Gibt die Höhe=Breite der Eck-Kanten an
velem = border_left.getHeight(), // Gibt die Höhe eines vertikalen Elements an
vborder_width = border_left.getWidth(), // Gibt die Breite eines vertikalen Rands an
vframe_width = frame_left.getWidth(), // Gibt die Breite eines vertikalen Rahmens an
helem = border_down.getWidth(), // Gibt die Breite eines horizontalen Elements an
hborder_height = border_down.getHeight(), // Gibt die Höhe eines horizontalen Rands an
bg_width = img_background.getWidth(), // Gibt die Breite des Hintergrundbildes an
bg_height = img_background.getHeight(), // Gibt die Höhe des Hintergrundbildes an
att_width = img_attention.getWidth(), // Gibt die Breite des Attentionbildes an
att_height = img_attention.getHeight(), // Gibt die Höhe des Attentionbildes an
client_width = width - 2 * BORDER, // Gibt die Client-Breite an (ohne Grenzen)
client_height = height - 2 * BORDER, // Gibt die Client-Höhe an (ohne Grenzen)
effective_width = width - 2 * edge, // Gibt die effektive-Breite an (ohne Kanten)
effective_height = height - 2 * edge, // Gibt die effektive-Höhe an (ohne Kanten)
// f(x) = ((x-1)/2)*2+1 calculates, how many hcomponents are placed.. Number is always odd!
hcount = ((effective_width - helem) / (2 * helem)) * 2 + 1,
// Gibt an, wieviele horizontale Elemente nebeneinander passen
vcount = effective_height / velem,
// Gibt an, wieviele vertikale Elemente nebeneinander passen
hindent = (effective_width - hcount * helem) / 2;
// Gibt die Einrückung der horizontalen Rahmenleiste an, damit sie mittig liegt
int x = client_width / bg_width,
y = client_height / bg_height;
int dx = client_width - (x * bg_width), dy = client_height - (y * bg_height);
BufferedImage xcomp = null, ycomp = null, missing = null;
// Schleife, um AttentionBar zu zeichnen..
x = client_width / att_width;
dx = client_width - (x * att_width);
xcomp = null;
if (dx > 0 ) {
xcomp = img_attention.getSubimage(0, 0, dx, att_height);
}
for (int i = 0; i <= x; i++) {
if (i == x && xcomp != null) {
g.drawImage(xcomp, i * att_width + BORDER, BORDER, null);
}
else {
g.drawImage(img_attention, i * att_width + BORDER, BORDER, null);
}
}
// Obere und Untere Begrenzung zeichnen..
toggle = true;
for (int i = 0; i < hcount; i++) {
x = i * velem + edge;
if (toggle) {
// Draw Top Border..
g.drawImage(border_top, x + hindent, 0, null);
}
else {
// Draw Top Frame
g.drawImage(frame_top, x + hindent, 0, null);
}
toggle = !toggle;
// Draw Limiter between Attention- and Client-Section..
g.drawImage(border_down, x, att_height + BORDER, null);
// Draw Bottom Border..
g.drawImage(border_down, x, height - hborder_height, null);
}
x = edge + effective_width - helem;
g.drawImage(border_top, edge, 0, null);
g.drawImage(border_top, x, 0, null);
g.drawImage(border_down, x - helem, height - hborder_height, null);
g.drawImage(border_down, x, height - hborder_height, null);
g.drawImage(border_down, x, att_height + BORDER, null);
g.drawImage(border_down, x - helem, att_height + BORDER, null);
// Linke und Rechte Begrenzung zeichnen..
// TODO: Draw from Bottom to Top, therefore in the attention-area can normal borders be placed..
toggle = true;
for (int j = 1; j <= vcount; j++) {
y = height - j * velem - edge;
if (toggle || j == vcount) {
// Draw Left Border..
g.drawImage(border_left, 0, y, null);
// Draw Right Border..
g.drawImage(border_right, width - vborder_width, y, null);
}
else {
// Draw Left Frame..
g.drawImage(frame_left, 0, y, null);
// Draw Right Frame..
g.drawImage(frame_right, width - vframe_width, y, null);
}
toggle = !toggle;
}
g.drawImage(border_left, 0, edge, null);
g.drawImage(border_right, width - edge + 1, edge, null);
// Setzen der 4 Ecken..
x = width - edge;
y = height - edge;
g.drawImage(node_topleft, 0, 0, null);
g.drawImage(node_topright, x, 0, null);
g.drawImage(node_downleft, 0, y, null);
g.drawImage(node_downright, x, y, null);
}
public Insets getBorderInsets(Component comp) {
return new Insets(50, 20, 20, 20);
}
public boolean isBorderOpaque() {
return false;
}
}
private static boolean initialized = false;
private static BufferedImage border_down, border_left, border_top, border_right, frame_top,
frame_left, frame_right, node_downleft, node_downright, node_topleft, node_topright,
img_background, img_attention;
private final boolean attention;
private int height, width;
public BasicDialog(int width, int height, boolean attention) {
if (!initialized) {
border_down = ImageManager.addImage("border_south", "System/Frame/border_down.png");
border_left = ImageManager.addImage("border_west", "System/Frame/border_left.png");
border_right = ImageManager.addImage("border_east", "System/Frame/border_right.png");
border_top = ImageManager.addImage("border_north", "System/Frame/border_top.png");
frame_top = ImageManager.addImage("frame_north", "System/Frame/frame_top.png");
frame_left = ImageManager.addImage("frame_west", "System/Frame/frame_left.png");
frame_right = ImageManager.addImage("frame_east", "System/Frame/frame_right.png");
node_downleft = ImageManager.addImage("south_west", "System/Frame/node_downleft.png");
node_downright = ImageManager.addImage("south_east", "System/Frame/node_downright.png");
node_topleft = ImageManager.addImage("north_west", "System/Frame/node_topleft.png");
node_topright = ImageManager.addImage("north_east", "System/Frame/node_topright.png");
img_background = ImageManager.addImage("foreground", "sterne-blue.bmp");
img_attention = ImageManager.addImage("attention", "System/attention.bmp");
initialized = true;
}
setBackground(new Color(0, 0, 0, 0));
setBorder(new AttentionBorder());
this.height = height;
this.width = width;
this.attention = attention;
String name = "bladeline";
setFont(Font.decode(name + "-24"));
this.setSize(width, height);
this.setLocation(
Toolkit.getDefaultToolkit().getScreenSize().width / 2 - width / 2,
Toolkit.getDefaultToolkit().getScreenSize().height / 2 - height / 2);
// Buttons erzeugen..
setLayout(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
JButton btn_cancel = new JButton("Cancel");
JButton btn_continue = new JButton("Continue");
btn_continue.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
Screenshot.execute();
}
});
c.insets = new Insets(0,30,25,0);
c.gridx = 0;
c.gridy = 1;
c.weighty = 1.0;
c.weightx = 1.0;
c.anchor = GridBagConstraints.SOUTHWEST;
add(btn_cancel, c);
c.insets = new Insets(0,0,25,30);
c.gridx = 1;
c.gridy = 1;
c.weighty = 1.0;
c.weightx = 1.0;
c.anchor = GridBagConstraints.SOUTHEAST;
add(btn_continue, c);
// Label erzeugen..
JLabel label = new JLabel();
label.setText("Lorem Ipsum dolorit");
Font font = getFont();
label.setFont(font);
label.setForeground(new Color(127, 127, 255));
c.insets = new Insets(10,10,10,10);
c.gridx = 0;
c.gridy = 1;
c.weighty = 1.0;
c.weightx = 1.0;
c.anchor = GridBagConstraints.CENTER;
add(label, c);
repaint();
setVisible(true);
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (attention) {
paintAttention(g);
}
else {
paintNonAttention(g);
}
}
protected void paintBorder(Graphics g) {
}
private void paintAttention(Graphics g) {
// TODO: Enforce Border do be top and down, and frame to be embedded.. then easily
// overwriting the image can be done without creating new subimages..
boolean toggle;
final int BORDER = 10, // Gibt die Höhe=Breite der Grenzen an
edge = node_topleft.getHeight(), // Gibt die Höhe=Breite der Eck-Kanten an
velem = border_left.getHeight(), // Gibt die Höhe eines vertikalen Elements an
vborder_width = border_left.getWidth(), // Gibt die Breite eines vertikalen Rands an
vframe_width = frame_left.getWidth(), // Gibt die Breite eines vertikalen Rahmens an
helem = border_down.getWidth(), // Gibt die Breite eines horizontalen Elements an
hborder_height = border_down.getHeight(), // Gibt die Höhe eines horizontalen Rands an
bg_width = img_background.getWidth(), // Gibt die Breite des Hintergrundbildes an
bg_height = img_background.getHeight(), // Gibt die Höhe des Hintergrundbildes an
att_width = img_attention.getWidth(), // Gibt die Breite des Attentionbildes an
att_height = img_attention.getHeight(), // Gibt die Höhe des Attentionbildes an
client_width = width - 2 * BORDER, // Gibt die Client-Breite an (ohne Grenzen)
client_height = height - 2 * BORDER, // Gibt die Client-Höhe an (ohne Grenzen)
effective_width = width - 2 * edge, // Gibt die effektive-Breite an (ohne Kanten)
effective_height = height - 2 * edge, // Gibt die effektive-Höhe an (ohne Kanten)
// f(x) = ((x-1)/2)*2+1 calculates, how many hcomponents are placed.. Number is always odd!
hcount = ((effective_width - helem) / (2 * helem)) * 2 + 1,
// Gibt an, wieviele horizontale Elemente nebeneinander passen
vcount = effective_height / velem,
// Gibt an, wieviele vertikale Elemente nebeneinander passen
hindent = (effective_width - hcount * helem) / 2;
// Gibt die Einrückung der horizontalen Rahmenleiste an, damit sie mittig liegt
// Schleife um gesamten Hintergrund zu zeichnen..
int x = client_width / bg_width,
y = client_height / bg_height;
int dx = client_width - (x * bg_width), dy = client_height - (y * bg_height);
BufferedImage xcomp = null, ycomp = null, missing = null;
if (dx > 0 && dy > 0) {
xcomp = img_background.getSubimage(0, 0, dx, bg_height);
ycomp = img_background.getSubimage(0, 0, bg_width, dy);
int xmissing = client_width - (client_width / bg_width) * bg_width;
int ymissing = client_height - (client_height / bg_height) * bg_height;
if (xmissing > 0 && ymissing > 0) missing = img_background.getSubimage(0, 0, xmissing, ymissing);
}
for (int i = 0; i <= x; i++) {
for (int j = 0; j <= y; j++) {
if (i == x && j == y && missing != null) {
// Last
g.drawImage(missing, BORDER + bg_width * i, BORDER + bg_height * j, null);
}
else if (i == x && xcomp != null) {
// xcomp
g.drawImage(xcomp, BORDER + bg_width * i, BORDER + bg_height * j, null);
}
else if (j == y && ycomp != null) {
// ycomp
g.drawImage(ycomp, BORDER + bg_width * i, BORDER + bg_height * j, null);
}
else {
// Standard Fields..
g.drawImage(img_background, BORDER + bg_width * i, BORDER + bg_height * j, null);
}
}
}
// Schleife, um AttentionBar zu zeichnen..
x = client_width / att_width;
dx = client_width - (x * att_width);
xcomp = null;
if (dx > 0 ) {
xcomp = img_attention.getSubimage(0, 0, dx, att_height);
}
for (int i = 0; i <= x; i++) {
if (i == x && xcomp != null) {
g.drawImage(xcomp, i * att_width + BORDER, BORDER, null);
}
else {
g.drawImage(img_attention, i * att_width + BORDER, BORDER, null);
}
}
// Obere und Untere Begrenzung zeichnen..
toggle = true;
for (int i = 0; i < hcount; i++) {
x = i * velem + edge;
if (toggle) {
// Draw Top Border..
g.drawImage(border_top, x + hindent, 0, null);
}
else {
// Draw Top Frame
g.drawImage(frame_top, x + hindent, 0, null);
}
toggle = !toggle;
// Draw Limiter between Attention- and Client-Section..
g.drawImage(border_down, x, att_height + BORDER, null);
// Draw Bottom Border..
g.drawImage(border_down, x, height - hborder_height, null);
}
x = edge + effective_width - helem;
g.drawImage(border_top, edge, 0, null);
g.drawImage(border_top, x, 0, null);
g.drawImage(border_down, x - helem, height - hborder_height, null);
g.drawImage(border_down, x, height - hborder_height, null);
g.drawImage(border_down, x, att_height + BORDER, null);
g.drawImage(border_down, x - helem, att_height + BORDER, null);
// Linke und Rechte Begrenzung zeichnen..
// TODO: Draw from Bottom to Top, therefore in the attention-area can normal borders be placed..
toggle = true;
for (int j = 1; j <= vcount; j++) {
y = height - j * velem - edge;
if (toggle || j == vcount) {
// Draw Left Border..
g.drawImage(border_left, 0, y, null);
// Draw Right Border..
g.drawImage(border_right, width - vborder_width, y, null);
}
else {
// Draw Left Frame..
g.drawImage(frame_left, 0, y, null);
// Draw Right Frame..
g.drawImage(frame_right, width - vframe_width, y, null);
}
toggle = !toggle;
}
g.drawImage(border_left, 0, edge, null);
g.drawImage(border_right, width - edge + 1, edge, null);
// Setzen der 4 Ecken..
x = width - edge;
y = height - edge;
g.drawImage(node_topleft, 0, 0, null);
g.drawImage(node_topright, x, 0, null);
g.drawImage(node_downleft, 0, y, null);
g.drawImage(node_downright, x, y, null);
}
private void paintNonAttention(Graphics g) {
// Not implemented yet
}
public boolean hasAttention() {
return attention;
}
}
Zuletzt bearbeitet: