Hallo zusammen - Ich hänge gerade an folgendem:
Die Klasse FixedSizeTable erbt von der Klasse Table und hat folgende Instanzvariablen:
Mein aktueller Code sieht so aus:
Dabei entstehen jetzt noch folgende zwei Fehlermeldungen:
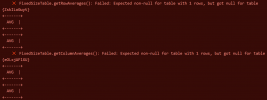
Ich bekomme das Problem leider einfach nicht gelöst - hat jemand einen Tipp für mich, was ich falsch mache bzw. wie ich den Fehler lösen kann?
LG Euler
Klasse FixedSizeTable
Implementieren Sie basierend auf der Klasse Table eine weitere Klasse FixedSizeTable, die eine Tabelle mit einer fixen Anzahl an Einträgen. Wenn eine solche Tabelle vollständig befüllt ist, dann können keine neuen Einträge mehr eingefügt werden.Die Klasse FixedSizeTable erbt von der Klasse Table und hat folgende Instanzvariablen:
- capacity: Die maximale Anzahl der Einträge der Tabelle.
- size: Die Anzahl der tatsächlichen Einträge der Tabelle.
- rows: Die Einträge der Tabelle als Row-Array.
Mein aktueller Code sieht so aus:
Java:
import java.util.Arrays;
public class FixedSizeTable extends Table {
private int capacity;
private int size;
private Row[] rows;
/**
* Create a new fixed-size table.
*
* @param name The name of the table
* @param capacity How many rows this table should store.
*/
public FixedSizeTable(String name, int capacity) {
super(name);
this.capacity = capacity;
this.size = 0;
this.rows = new Row[capacity];
}
/**
* Returns the capacity of the table.
*/
public int getCapacity() {
return capacity;
}
/**
* Returns the number of rows stored in the table.
*/
public int getSize() {
return size;
}
/**
* Returns the rows stored in the table.
*/
public Row[] getRows() {
return rows;
}
/**
* Sets the number of rows stored in the table.
*/
public void setSize(int size) {
this.size = size;
}
/**
* Returns the row at the given index.
*
* @param index The index of the row to return.
* @return The row at the given index or null if the index is out of bounds.
*/
public Row getRow(int index) {
if (index < 0 || index >= capacity) {
return null;
}
return rows[index];
}
/**
* Sets the row at the given index.
*
* @param index The index of the row to set.
* @param row The new value of the row.
* @return true if the row was set, false otherwise, including if the index
* is out of bounds.
*/
public boolean setRow(int index, Row row) {
if (index < 0 || index >= capacity) {
return false;
}
rows[index] = row;
return true;
}
/**
* Resizes the table.
*
* If the new size is smaller than the old size, the table is truncated,
* i.e. all rows with an index >= capacity are deleted; if the new size
* is larger than the old size, the table is extended by adding new rows
* that are initialized with `null`.
*
* @param capacity The new capacity of the table.
* @return The new capacity of the table.
*/
public int resize(int capacity) {
if (capacity < this.capacity) {
size = Math.min(size, capacity);
}
Row[] newRows = new Row[capacity];
System.arraycopy(rows, 0, newRows, 0, Math.min(rows.length, capacity));
rows = newRows;
if (capacity > this.capacity) {
for (int i = this.capacity; i < capacity; i++) {
rows[i] = null;
}
}
this.capacity = capacity;
return this.capacity;
}
/**
* Adds a row to the table.
*
* @param row The row to add.
* @return true if the row was added, false otherwise, i.e. if the table
* is full.
*/
public boolean addRow(Row row) {
if (size < capacity) {
rows[size] = row;
size++;
return true;
}
return false;
}
/**
* Returns the maximum dimension amongst rows in the table.
*
* The dimension of a `null` row is 0.
*/
public int getMaxDimension() {
if (rows == null || rows.length == 0) {
return 0;
}
int maxDimension = 0;
for (Row row : rows) {
if (row != null && row.getDimension() > maxDimension) {
maxDimension = row.getDimension();
}
}
return maxDimension;
}
/**
* Returns the average of each row.
*
* Empty (i.e. NaN) values are excluded from the calculation entirely.
*
* @return If the table is not empty, a new array containing the
* average of each row or 0 if a row is `null`. If the table is empty,
* `null` is returned. If all entries are NaN, NaN is returned.
*/
public double[] getRowAverages() {
if (size == 0 || rows == null || rows.length == 0) {
return null;
}
double[] averages = new double[size];
for (int i = 0; i < size; i++) {
if (rows[i] == null) {
averages[i] = 0; // Wenn die Zeile null ist, setze den Durchschnitt auf 0
} else {
averages[i] = rows[i].getAverage(); // Andernfalls berechne den Durchschnitt der Zeile
}
}
return averages;
}
/**
* Returns the average of each column.
*
* Empty (i.e. NaN) values are excluded from the calculation entirely.
*
* @return If the table is not empty, a new array containing the
* average of each column or 0 if a row is `null`. If the table
* is empty, `null` is returned. If all entries are NaN, NaN is returned.
*/
public double[] getColumnAverages() {
if (size == 0 || rows == null) {
return null;
}
int maxDimension = getMaxDimension();
double[] columnSums = new double[maxDimension];
int[] countNaN = new int[maxDimension];
for (Row row : rows) {
if (row != null) {
double[] rowData = row.getValues();
for (int i = 0; i < rowData.length; i++) {
if (!Double.isNaN(rowData[i])) {
columnSums[i] += rowData[i];
countNaN[i]++;
}
}
}
}
double[] columnAverages = new double[maxDimension];
for (int i = 0; i < maxDimension; i++) {
columnAverages[i] = (countNaN[i] > 0) ? columnSums[i] / countNaN[i] : Double.NaN;
}
return columnAverages;
}
/**
* Returns a string representation of the table.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Table Name: ").append(getName()).append("\n");
// Header row for column numbers
sb.append(" ");
for (int i = 0; i < getMaxDimension(); i++) {
sb.append("V").append(String.format("%02d", i)).append(" ");
}
sb.append("\n");
for (int i = 0; i < size; i++) {
sb.append("R").append(String.format("%02d", i)).append(": ");
Row currentRow = rows[i];
if (currentRow != null) {
sb.append(currentRow.toString());
} else {
sb.append("Empty row");
}
sb.append("\n");
}
// Row averages
double[] rowAverages = getRowAverages();
if (rowAverages != null) {
sb.append("Row Averages: ");
for (double average : rowAverages) {
sb.append(String.format("%.3f", average)).append(" ");
}
sb.append("\n");
}
// Column averages
double[] columnAverages = getColumnAverages();
if (columnAverages != null) {
sb.append("Column Averages: ");
for (double average : columnAverages) {
sb.append(String.format("%.3f", average)).append(" ");
}
sb.append("\n");
}
return sb.toString();
}
// Ignore this. It’s here for the tests to work properly.
protected FixedSizeTable() {}
}
Dabei entstehen jetzt noch folgende zwei Fehlermeldungen:
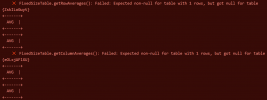
Ich bekomme das Problem leider einfach nicht gelöst - hat jemand einen Tipp für mich, was ich falsch mache bzw. wie ich den Fehler lösen kann?
LG Euler